Push notifications are one of the best ways to engage users on your website or app by delivering timely information on updates. But how to implement them?
In this guide, we’ll delve into how developers can implement push notifications with Django environment using technologies like Celery and Redis.
Pre-requisites
Before implementing push notifications, ensure the following prerequisites are in place:
- A functioning Django environment
- Installation of necessary dependencies, including Celery, Pyfcm, A message broker (e.g., Redis) supported by Celery, along with the Django-redis backend client, and pans (Note: Requires an older version of Python for iOS notifications support).
- Familiarity with Firebase for obtaining the Firebase API Token.
- Acquiring an APNS certificate and understanding its configuration.
- Understanding the concept of device tokens, essential for identifying user devices.
Steps to Implement Push Notifications with Django Using Celery and Redis
You can follow these simple steps to implement push notification with Django –
Set up the Django project
If you haven’t already, create a Django project by running
django-admin startproject project_name
Install required packages
Install the necessary packages for Celery, Redis, and push notifications.
pip install django celery redis django-celery-beat
Install the necessary packages for Celery, Redis, and push notifications.
pip install django celery redis django-celery-beat
Configure Celery
Add Celery configuration to your Django settings.
# settings.py
CELERY_BROKER_URL = 'redis://localhost:6379/0'
CELERY_RESULT_BACKEND = 'redis://localhost:6379/0'
Create Celery tasks
Define tasks that will handle sending push notifications.
For example:
# tasks.py
from celery import shared_task
from push_notifications.models import APNSDevice, GCMDevice
@shared_task
def send_push_notification(device_id, message):
# Retrieve device and send push notification
device = APNSDevice.objects.get(id=device_id)
device.send_message(message)
Create a model for push tokens
Create a model to store device tokens and associate them with users.
# models.py
from django.db import models
from push_notifications.models import APNSDevice, GCMDevice
class PushToken(models.Model):
user = models.ForeignKey(User, on_delete=models.CASCADE)
apns_token = models.CharField(max_length=255, blank=True, null=True)
gcm_token = models.CharField(max_length=255, blank=True, null=True)
Register devices
Whenever a user registers or logs in, register their device token.
from push_notifications.models import APNSDevice, GCMDevice
def register_device(user, token, platform):
if platform == 'ios':
APNSDevice.objects.create(user=user, registration_id=token)
elif platform == 'android':
GCMDevice.objects.create(user=user, registration_id=token)
Send push notifications
Trigger push notifications using Celery tasks whenever necessary.
from .tasks import send_push_notification
def send_notification_to_user(user_id, message):
user = User.objects.get(pk=user_id)
push_token = PushToken.objects.get(user=user)
if push_token.apns_token:
send_push_notification.delay(push_token.apns_token, message)
if push_token.gcm_token:
send_push_notification.delay(push_token.gcm_token, message)
Run Celery worker
Start a Celery worker to process tasks.
celery -A your_project_name worker -l info
Test push notifications
Test sending push notifications to your registered devices to ensure everything is working as expected.
Remember to replace your_project_name with the name of your Django project and adjust the code according to your specific requirements. Additionally, make sure to configure Redis and any additional settings required by your push notification service provider.
Too much coding? There’s an easier way!
What if you are not a developer yet and still want to send push notifications and drive traffic?
Well, there’s a way better solution for bloggers, publishers and business owners. You can use the push notifications service to send notifications without having to worry about all these codings.
You might be wondering, why pay for a service when you can implement these for free?
Here are the reasons why you should go for a service provider instead of implementing the code yourself.
Time and Resource Efficiency
By opting for a service provider, users bypass the time-consuming setup involved in coding notifications from scratch. These providers streamline the process, enabling quick initiation of notification campaigns.
This efficiency saves valuable time and resources that would otherwise be spent on managing servers, databases, and infrastructure, allowing users to focus more on content creation and engagement strategies.
Advanced Features for Enhanced Engagement
Push notification service providers offer a suite of advanced features that empower users to elevate their engagement strategies.
These features include audience segmentation, allowing tailored notifications based on demographics or user behavior.
Automation options AutoMagic Push enables setting up campaigns triggered by specific events, and A/B testing functionalities enable testing different notification formats or timings, optimizing engagement rates without manual intervention.
User-Friendly Interface
Push notification service providers offer intuitive dashboards and user interfaces that simplify the setup process.
These interfaces are designed for non-technical users, enabling easy navigation and allowing anyone to create and send notifications without requiring coding skills.
Users can quickly grasp the platform’s functionalities, making it accessible for bloggers, publishers, and businesses alike.
Cross-Platform Support
One of the notable benefits of service providers is their ability to reach users across multiple platforms seamlessly. They facilitate communication with audiences on various devices, including mobile, desktop, and web browsers.
This broad reach ensures consistent messaging and engagement across different channels, enhancing the overall user experience.
Analytics and Insights
Service providers offer robust analytics tools that provide valuable insights into notification performance. These analytics track open rates, click-through rates, and user engagement.
Leveraging this data allows users to make informed decisions, refine notification strategies, and tailor content for better audience engagement and retention.
Reliability and Compliance
Relying on the infrastructure provided by service providers ensures prompt and reliable delivery of notifications.
Moreover, these providers often handle compliance with industry standards and regulations, such as GDPR, ensuring legal adherence and data protection and providing peace of mind for users concerned with regulatory requirements.
Dedicated Customer Support
Push notification service providers typically offer dedicated customer support. This support includes technical assistance for troubleshooting, guidance on using LaraPush’s features effectively, and regular updates and maintenance.
Users can rely on expert support teams for any issues or queries, ensuring a smooth experience while using the service.
While coding may seem cost-effective initially, considering time, resources, and potential complexities, a service provider offers scalable and affordable pricing plans tailored to usage and audience size.
Best Push Notification Service Provider: LaraPush
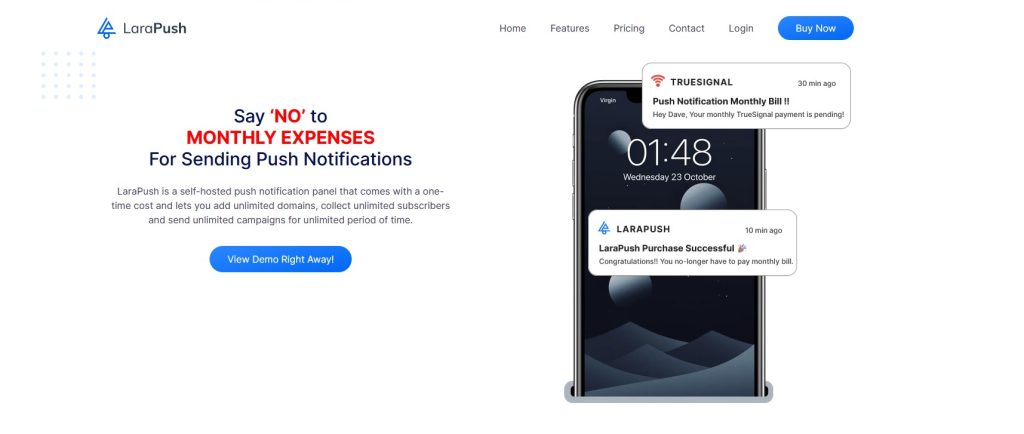
We would say LaraPush.
Let’s put the pricing aside for a while. We provide the most affordable services, with unlimited domains, subscribers, and campaigns for lifetime with just a single one-time payment.
And as our clients say they have saved the most with LaraPush and we will tell you how later on.
But buying a service just because it’s affordable is too big of a risk to take. Hence, we are here to walk you through the value LaraPush provides to its users.
- WordPress Integration: This feature allows you to easily send push notifications to your audience directly from your WordPress site. You can instantly notify your subscribers whenever you publish new content, boosting engagement without any hassle.
- On-Time Delivery: On-time delivery, with perfect accuracy, determines the success of your push notification campaigns. If you miss it by a few minutes, your audience may not have time to look at the notification or find it irritating. LaraPush understands the importance of on-time delivery. We deliver you messages at the scheduled time, ensuring high CTR.
- Analytics Insights: Understanding your audience, like their location, preferences, behavior, etc., helps you build an effective notification strategy. You can use these insights to create more targeted campaigns and content that resonate better with your audience.
- Customizable Prompt: You can design the subscription prompt to match your website’s look and feel, providing a seamless user experience and maintaining your brand’s consistency.
- AMP Compatibility: Being AMP-compatible means your content can load faster on mobile devices, improving visibility on Google and making it easier for users to subscribe to your notifications.
- AutoMagic Push and Push On Publish: Auto Magic saves your time by automating your push notifications. For example, Let’s say you want to send a notification on an interval of 12 hours; you can completely automate the process without having to log in every 12 hours.
What’s the deal for LaraPush?
You might be wondering, too many features come with too high a price.
Absolutely not! Kind of the opposite. You get all these features with a lifetime deal.
You can choose from two pricing plans: the STARTUP at $499 or the PRO at $799, both on a one-time payment model.
Conclusion
The process of implementing push notifications within Django involves a series of interconnected steps, integrating various tools and technologies to achieve real-time notification delivery.
By following this comprehensive guide, developers can gain a profound understanding of each phase involved, empowering them to seamlessly incorporate push notification functionality into their Django-based applications.
Remember, while the detailed approach works perfectly, you can simply choose a service provider like LaraPush and start sending notifications right away.